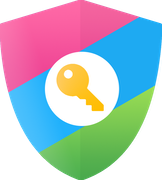
NextAuth.js
Authentication for Next.js
Open Source. Full Stack. Own Your Data.
Flexible
- Built for Serverless, runs anywhere
- Bring Your Own Database - or none!
(MySQL, Postgres, MongoDB…) - Choose Database Sessions or JWT
- Secure web pages and API routes
Easy
- Built in support for popular services
(Google, Facebook, Auth0, Apple…) - API for OAuth service integration
- Email / Passwordless / Magic Link
- Use any username/password store
Secure
- Signed, prefixed, server-only cookies
- CSRF Tokens on HTTP POST
- JWT with JWS / JWE / JWK / JWK
- Tab Sync, Auto Revalidation, Keepalive
- Doesn't rely on client side JavaScript
Add authentication in minutes with ready made example code.
Server
import NextAuth from 'next-auth'
import Providers from 'next-auth/providers'
const options = {
providers: [
// OAuth authentication providers
Providers.Apple({
clientId: process.env.APPLE_ID,
clientSecret: process.env.APPLE_SECRET
}),
Providers.Facebook({
clientId: process.env.FACEBOOK_ID,
clientSecret: process.env.FACEBOOK_SECRET
}),
Providers.Google({
clientId: process.env.GOOGLE_ID,
clientSecret: process.env.GOOGLE_SECRET
}),
// Sign in with email
Providers.Email({
server: process.env.MAIL_SERVER,
from: 'NextAuth.js <no-reply@example.com>'
}),
],
// MySQL, Postgres or MongoDB database (or leave empty)
database: process.env.DATABASE_URL
}
export default (req, res) => NextAuth(req, res, options)
Client
import React from 'react'
import {
signIn,
signOut,
useSession
} from 'next-auth/client'
export default function myComponent() {
const [ session, loading ] = useSession()
return <>
{!session && <>
Not signed in <br/>
<button onClick={signIn}>Sign in</button>
</>}
{session && <>
Signed in as {session.user.email} <br/>
<button onClick={signOut}>Sign out</button>
</>}
</>
}
NextAuth.js is not affiliated with Vercel or Next.js